In a previous post, Install Team Explorer Everywhere 2010, I covered the installation of TEE, so now I’m going to cover connecting to a TFS team project collection.
1. To get started open the Team Foundation Server Exploring (TFS Explorer for short) perspective, this can be found in Window ^ Open Perspective ^ Other…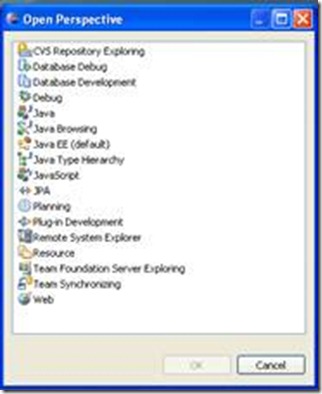
Now your current windows should look like the screen shown below. The icon used to add a TFS server connection is circled in red, which will be used in the next step. If you’ve used TFS with Visual Studio, you’ll notice that the layout is nearly identical to what it is in Visual Studio.
![clip_image002[7] clip_image002[7]](http://lh5.ggpht.com/_62SKYZzoLpk/TLCsqBIAMpI/AAAAAAAAAE0/VpH4GA77f8M/clip_image002%5B7%5D_thumb%5B3%5D.jpg?imgmax=800)
2. To add the TFS server, click on the icon that is circled in the previous image. The “Add Existing Team Project” dialog will appear. This is where you add your TFS server (see image below). The advanced tab allows you to port settings, for example if you’re using SSL. Enter in the correct server URL, the default would be http://{server}:8080/tfs. Click Next.
![clip_image002[9] clip_image002[9]](http://lh6.ggpht.com/_62SKYZzoLpk/TLCsryMaGQI/AAAAAAAAAE8/rYFZt_EAQg0/clip_image002%5B9%5D_thumb%5B3%5D.jpg?imgmax=800)
3. Continuing from the server setup, you should be on the Team Project selection page. On the left pane is the team project collections list. Prior to TFS 2010, there could only be one project collection. On the right pane are the team projects within the collection. Select the team projects that you want view. Click Next.
![clip_image002[11] clip_image002[11]](http://lh6.ggpht.com/_62SKYZzoLpk/TLCstQjgXsI/AAAAAAAAAFE/pUEkoFfuYHM/clip_image002%5B11%5D_thumb%5B1%5D.jpg?imgmax=800)
4. Once it finishes querying TFS, the last window will appear showing any workspaces you have. Here I have one because I already created a workspace through Visual Studio. Select your workspace and click “Finish” if you have one else continue with this step to create one.
.
If you don’t have a workspace defined, you can create one by using the “Add…” button. You will see the workspace dialog appear as shown below. By default the workspace name will be your machine name. In the working folder you must map a source folder to a local folder. In the Status column select Active from the options. In the Source Control Folder option, click the browse “…” icon to bring up the TFS source “Browse for Folder” dialog. Select the source path you want and click Ok. Now in the Local Folder column, select the browse “…” icon to pick local file path where you want the source to copy down to. Once done you can click Ok to finish creating workspace.
![clip_image002[15] clip_image002[15]](http://lh6.ggpht.com/_62SKYZzoLpk/TLCsxKvlz8I/AAAAAAAAAFU/iRTKmHckqxU/clip_image002%5B15%5D_thumb%5B2%5D.jpg?imgmax=800)
5. Now the Team Explorer should be filled in and some more sub-windows should appear (see image below). With a team project expanded double click the “Source Control” item, see red circled item. This will display the Source Control window. Below highlights the 3 main windows that I use on a regular basis.
- Team Explorer: Contains a list of team project collections, and under each collection is a list of team projects. Items will show up based on what you have selected in your workspace.
- Source Control: Shows a folder tree display of all available branches to you. Navigating these you will be able to see whatever you have checked in to these branches.
- Pending Changes: This shows a list of any pending changes that you have checked out. You can also check the items in from this pain as well, shelve/unshelve, associated check-in with work items, etc.
![clip_image002[17] clip_image002[17]](http://lh4.ggpht.com/_62SKYZzoLpk/TLCs0vwVaQI/AAAAAAAAAFc/SqXmpKnNZvE/clip_image002%5B17%5D_thumb%5B3%5D.jpg?imgmax=800)
And that’s it, you are now ready to check in/out your project files to TFS and take advantage of the integrated features of TFS such as bug tracking and shelving. Shelving is a neat feature of TFS that allows you place your code on a virtual “book shelf” with some name so at some later date you can retrieve this named “book” of code from the shelf. This is very useful if your working on a large feature and you have files checked out for more than a day. At the end of the day you can shelve the code, which stores your changes on the server. If your machines dies for some odd reason, all your hard work is not lost. You can also share you shelved items with other team members. For details of shelving see, Walkthrough: Shelving Version Control Items.